Type Promotion in C
Type promotion in C is a method to convert any variable from one datatype to another. C allows variables of different datatypes to be present in a single expression. There are different types of type conversions available in C. They are Implicit type conversion and Explicit type conversion.
Different types of type conversions are mentioned in the below image. Let us understand each conversion with an example as a part of this blog post.
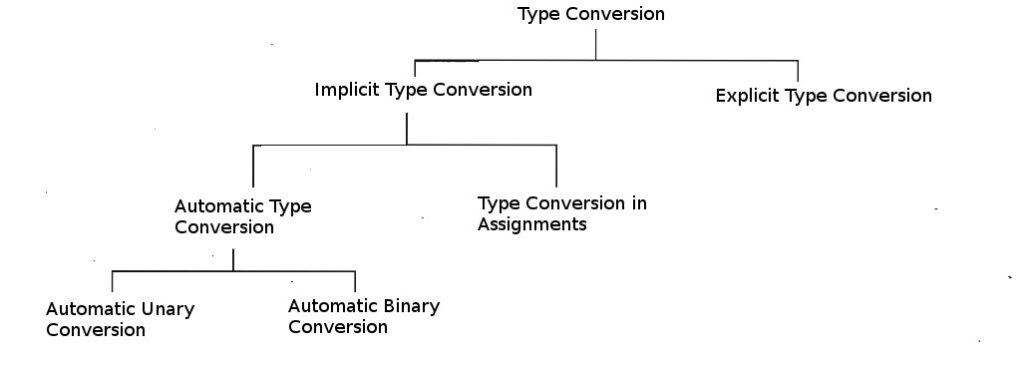
Implicit Type Conversions
Implicit type conversions are done by compilers. Implicit type conversions is also called as coercion. Only some programming languages allow compilers to provide implicit type conversion. These conversions are done by C compilers according to the predefined rules of C language. Here is an example to understand implicit type conversion.
Example
Code
Output

Automatic Unary and Binary Conversions
In the above given example, variable a is of type int and variable b is of type char. When addition is done in line 8 and stored in variable c and when we print it (line 9), we get the output as 98. ASCII value of variable ‘a’ is 97 added with 1.
Thus char variable b is implicitly type converted to integer. Automatic type conversion can be further split into unary and binary. Let us understand each one of the conversions with an example.
Unary
In any given expression, all operands of type char and type short will be converted into datatype int before any operation is performed. Some compilers convert float operand to double before any operation is performed. this is Automatic Unary Conversion.
Example
Code
Output

In the above given example, variable c is of type double and variable a is of type int. In line 11, variable a is implicitly converted into double and added with value 1.0. The final output is stored in variable c.
Binary
In any given expression, if one of the operands is long double or double or float or unsigned long int, the other operand will be converted into long double or double or float or unsigned long int respectively.
Example
Code
Output

In the given example, variable ‘a’ undergoes implicit type conversions based on the type of the destination variables. In line 12, ‘a’ is implicitly converted to unsigned int. In line 13, it is implicitly converted to float.
In line 14, it is implicitly converted to double. And in line 15, it is implicitly converted to long double. These conversions occur automatically to match the data types of the destination variables.
- In an expression, if one operand is long int and the other operand is unsigned int and if long int could hold all values of unsigned int, then the unsigned int variable will be converted into long int. Otherwise both the variables will be converted into unsigned long int.
- In an expression, if any one of the operands is long int or unsigned int the other variable will be converted into long int or unsigned int respectively.
- Otherwise both operands in an expression will be int and the result will also be int.
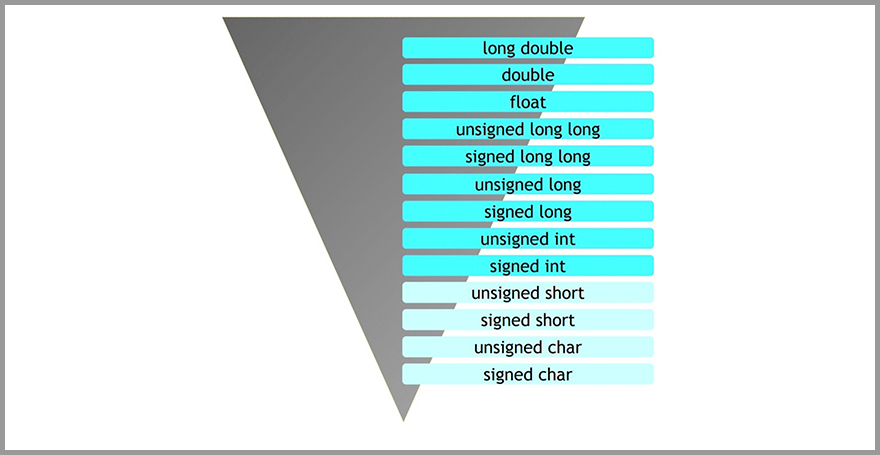
Whenever there are two operands of different data types in an expression, the operand with a lower rank will be converted to the datatype of higher rank operand.
Type Conversion In Assignment
Consider an assignment expression, where the types of two operands are different. The datatype of operand in Right Hand Side will be converted into datatype of operand in Left Hand Side. During this type conversion, either promotion or demotion of operand on right hand side.
Promotion
During datatype conversion, if operand on the right hand side is of lower rank type then it becomes the type of left hand side operand which is of higher rank. This kind of type conversion from lower rank to higher rank is called Promotion
Demotion
During datatype conversion, if operand on the right hand side is of higher rank type then it becomes the type of left hand side operand which is of lower rank. This kind of type conversion from higher rank to lower rank is called Demotion.
This promotion and demotion of type gives rise to some consequences. They are described below.
- Some high order bits may be dropped when long is converted to int, or int is converted to short int or char.
- Fractional part may be truncated during conversion of float type to int type.
- When double type is converted to float type, digits are rounded off.
- When a signed type is changed to unsigned type, the sign may be dropped.
- When an int is converted to float, or float to double there will be no increase in accuracy or precision.
Example
Code
Output

In the above given example, integer variable i_var1 and float variable f_var1 are demoted in line 12 and line 13, whereas in line 20 and line 21, integer variable i_var2 and character variable c_var2 are promoted to type float and int respectively.
Explicit Type Conversion or Type Casting
Generally in some cases, implicit type conversion will not solve the required purpose for which the expression is written. In that case, we could specify/write our own conversions. This is known as type casting/coercion.
Cast operator is an unary operator specially used for the purpose of converting an
expression to a particular datatype temporarily, where the expression can be of any constant or variable
Syntax
(datatype)expression
Example
Code
Output

In the given example, var1 and var2 are integer variables. When dividing var1 by var2 and storing the result in a float variable called “result,” the decimal values are truncated. However, by using a type cast operator in line 10 and temporarily converting var1 to float, we can obtain the decimal values accurately.
The value of result1 becomes 2.35 due to the type cast operator. It’s important to note that var1 remains an integer throughout the program except for line 10.Let us understand some more examples of usage of cast operator here.
(int)100.23223
Here constant 20.3 is converted to integer type. Hence the fractional part is lost and final result will be 100.
(float)100/3
Here constant 100 is converted to float type, then it is divided by 3. The value obtained is 33.33.
(float)(100/3)
Here at first, 100 is divided by 3 and then the result is converted into float type. The result will be 33.00.
(double)(x +y -z)
Here in the above expression result of expression x+y-z is converted to double.
(double)x+y-z
Here in the above expression x is first converted to double and then used in expression.
Conclusion
Though there are many promotion and demotion of types occurs as an effect of type conversions, they are of great help while dealing with variables of different data types in an expression.
Especially in explicit type conversion, where a datatype change occurs temporarily only in expression where cast operator is used.
Happy learning !!!
Serial No | Related Blog Posts | Links |
---|---|---|
1. | The Future Scope of Embedded Systems : Trends and Predictions | Click Here |
2. | Online Free IoT Internship for Engineering Students | Free Certificates |2023 | Click Here |
3. | Emertxe Placements in Embedded Systems and Embedded IoT – 2022 (Jan-sept) Report | Click Here |
People Also Ask (PAA)
Data type promotion in C involves automatic conversion of data between different types during operations or expressions for compatibility and accuracy
Type promotion in C involves automatic conversion of data between different types during operations or expressions, following rules to ensure compatibility and accurate results