Introduction
In the realm of C programming, pointers play a pivotal role as invaluable tools that enable us to efficiently manage memory and manipulate data. However, the terminology surrounding pointers can sometimes become perplexing, especially when dealing with two fundamental concepts: “Constant Pointer” and “Pointer Constant.”
In this blog post, our primary focus is to elucidate the distinctions between Constant Pointers and Pointer Constants, shedding light on their unique definitions, practical applications, and how they wield influence over the behavior of our code.
By gaining a clear understanding of these disparities, developers can elevate their code-writing skills, crafting more dependable and efficient solutions while harnessing the full potential of pointers within their C code.
Grasping the nuances between a constant pointer and a pointer to constant proves to be pivotal for achieving mastery in C programming.
When working with C programming, it’s essential to understand various concepts, including enumeration. If you’re looking to deeper into this topic, consider reading our blog post on Enumeration in C.
What is a Constant Pointer?
A pointer that cannot change the address it is containing. In other words, we can say that once a constant pointer points to a variable then it cannot point to any other variable. In fact it’s the reverse of the pointer constant which we discussed in the previous section.
To put it simply, a constant pointer is a pointer in C programming that has a fixed memory address and cannot be reassigned to point to a different memory location after its initialization. In other words, once a constant pointer is assigned a memory address, it remains bound to that address throughout its lifetime.
1. Declaration of Constant Pointer
It can be declared by applying the const qualifier, before the variable name. By applying the const before the variable name it will make it a constant pointer.

Example :

2. Constant Pointer Illustration
Let us consider the code snippet, pretty much similar in the lines of what we saw in the pointer constant section above.
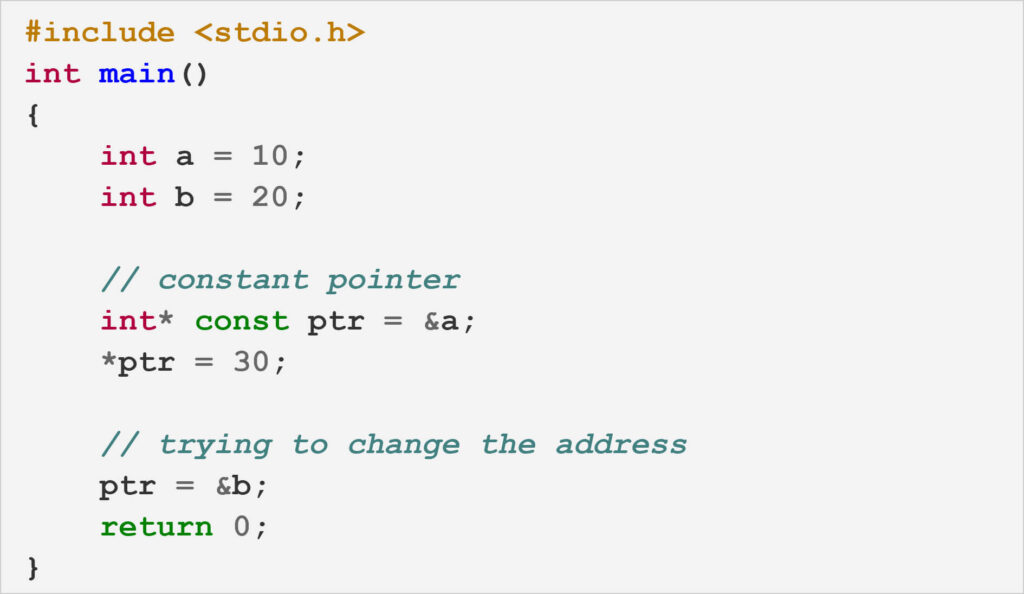
As you can see in the code, there are two variables a and b having values of 10 and 20 respectively. Now a constant pointer ptr is made to point to variable a. After making to point, the value of a can be changed via ptr by making *ptr = 30.
On the other hand ptr cannot be made to point to another address location of a variable b, hence this code will give an error.
Output :
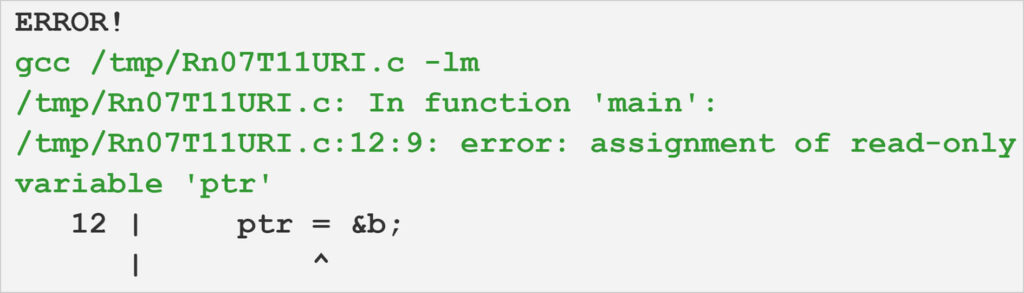
This can be clearly understood by the pictorial representation given below.
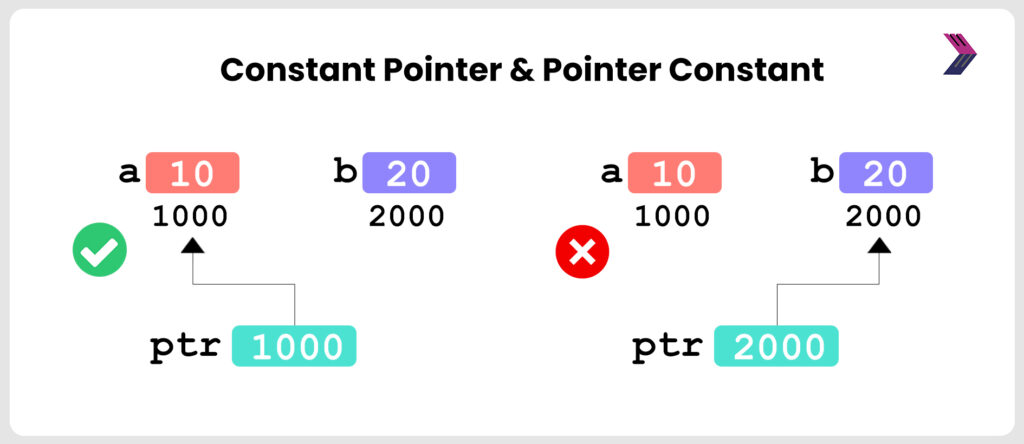
3. Constant Pointer - Summary
So the constant pointer can be understood as follows.
As the diagram illustrates, the value that the pointer points to can be changed. On the other hand the address the pointer points to can’t be changed.
This was illustrated in the code examples given above.

What is Pointer Constant ?
A constant pointer, also known as a pointer constant, is a term frequently employed in programming. It describes a pointer whose value, specifically the memory address it points to, remains unalterable following its initialization.
In simpler terms, once a constant pointer is assigned a memory address, it cannot be changed to reference a different memory location. This concept highlights the critical difference between a constant pointer and a pointer constant.
In many programming languages, such as C and C++, a constant pointer is declared by employing the const
keyword. This keyword guarantees that the memory address stored in the pointer remains immutable.
Notably, even if the data at the memory address is not marked as const
, it can still be modified, but the pointer to constant itself remains fixed in its original memory location.
1. Declaration of Pointer Constant
The pointer constant can be declared by applying the const qualifier, before the regular pointer declaration. By applying the const before the data type it will make it as a pointer constant.

Example :

2. Pointer Constant Illustration
Let us consider the code snippet below to understand how pointer constant works. As you can see there are two variables a and b along with a pointer constant named as ptr. The pointer constant is made to point to variable a by assigning its address.
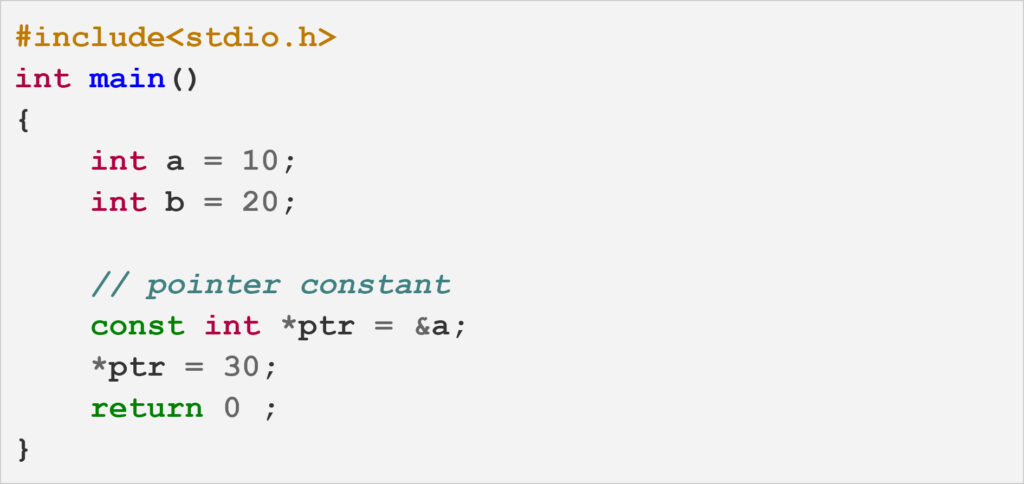
When you compile and run the code you will be getting a compilation error as follows.
Output :
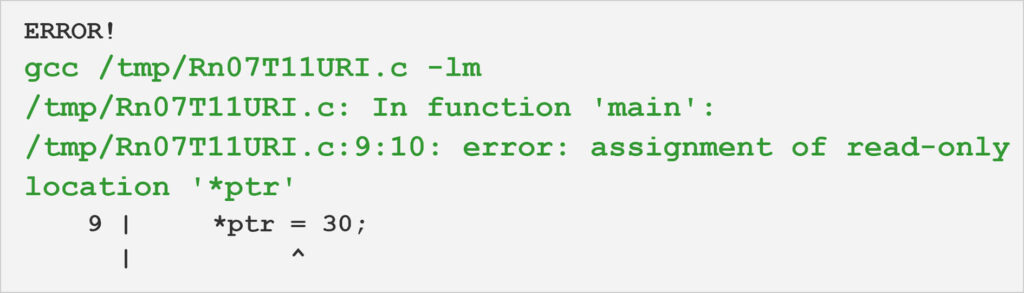
In the above given code, we have declared pointer constant ptr. Which means the data that is pointing to cannot be changed. Initially we assign it to point to the address of an integer variable a, say for example 1000.
Later when we try to alter it by assigning a constant value of 30 which is stored in a different location, it is not acceptable as per the pointer constant. Hence the compiler is giving an error.
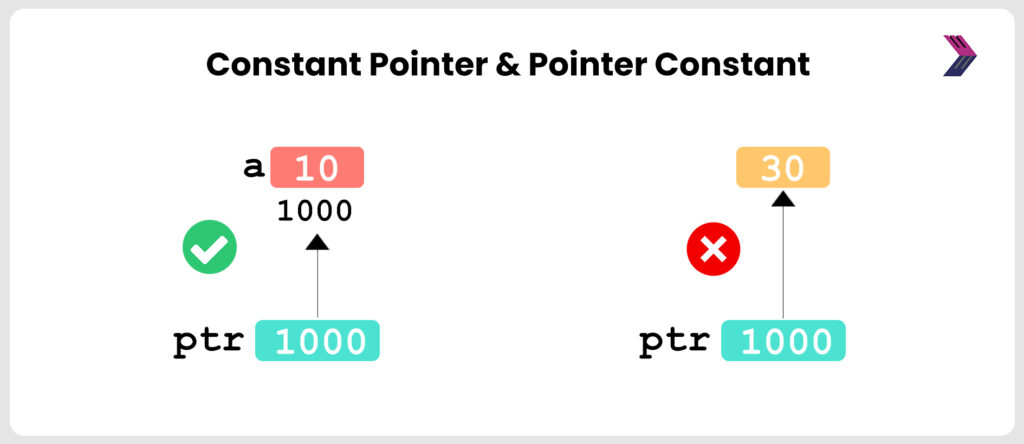
On the other hand, we can change the address of the pointer constant, where it is pointing to. Please refer to the code snippet given below.
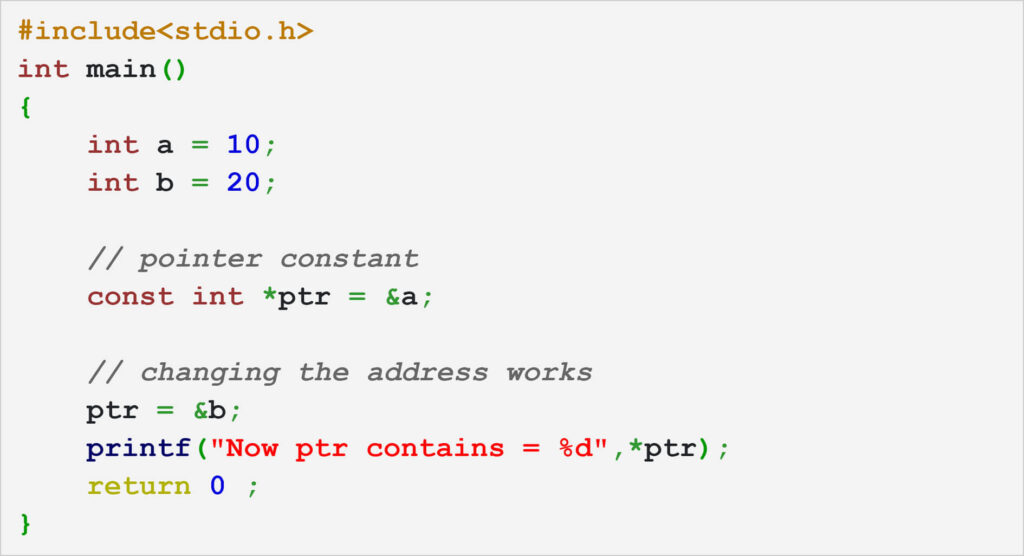
Let us consider two variables a and b having values of 10 and 20. Assume they are stored in address locations 1000 and 2000 in the memory respectively.
initially the pointer constant ptr is made to point to variable a, thereby having the value of 1000 inside the pointer variable ptr. Now the ptr can be modified to point to another variable b thereby having a value of 2000.
This means the address contained in the pointer constant can be changed from 1000 to 2000 but not the value it is pointing to.
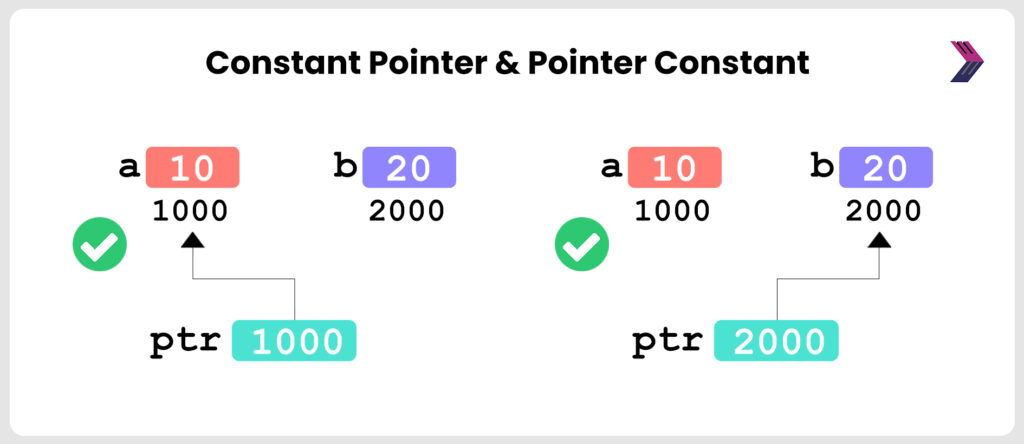
When you run the program you will get the proper output as given below. As you can see it gives a proper output as the address can be changed.
Output :

3. Pointer Constant - Summary
So the pointer constant can be understood as follows.
As the diagram illustrates, the value that the pointer points to can’t be changed. On the other hand the address the pointer points to can be changed.
This was illustrated in the code examples given above.

Pointer Constant - Example Applications
The pointer constant and constant pointer is used in many of the applications in C. One of the popular and well known examples is from the string library of C programming.
In popular string library functions strlen() and strcmp() is one such example. If you carefully examine the arguments getting passed to these functions, they use a pointer constant, as it needs to be passed without any attempt to modify the original string.
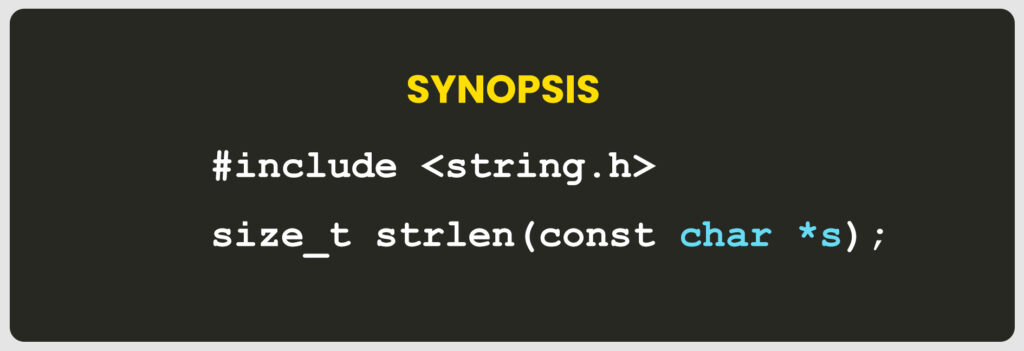
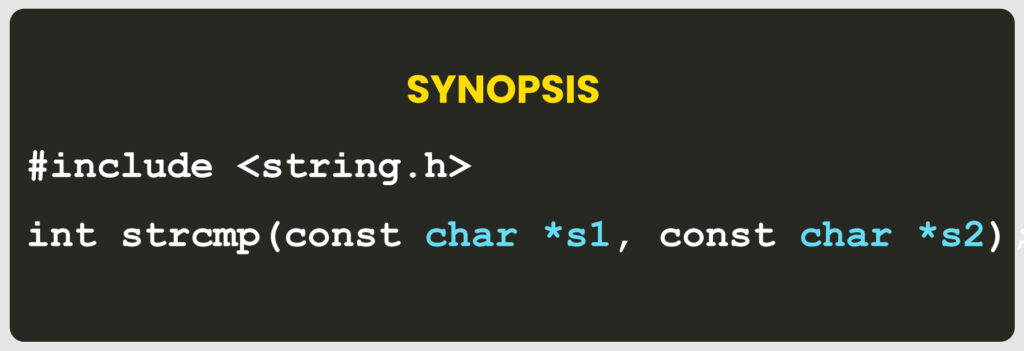
Pointer Constant V/S Constant Pointer - Comparison
In the realm of C programming, pointer constants and constant pointers are two concepts that often cause confusion due to their similar-sounding names. However, they have distinct meanings and implications in code. Let’s compare these two concepts side by side to understand their differences more clearly.
Aspect | Pointer Constant | Constant Pointer |
Definition | A pointer whose value (memory address) is constant. | A pointer with a fixed memory address. |
Declaration | data_type const *ptr; | data_type *const ptr; |
Value Modification | The pointer value (memory address) cannot be changed. | The pointer value (memory address) cannot be changed. |
Data Modification | The data at the memory address can be changed. | The data at the memory address can be changed. |
Pointer Reassignment | The pointer can be reassigned to point elsewhere. | The pointer cannot be reassigned to point elsewhere. |
Use Case | Useful when you want to prevent changing the pointer value while allowing data modification. | Useful when you need a stable pointer that always points to the same memory location. |
Given the syntax looks so similar, here is a simple table which you can keep handy to compare pointer constant and constant pointers.
Example | Part Before Asterisk | Part After Asterisk | Comments |
const char * ptr | const char | ptr | Const is associated with data type ,so value is constant |
const char * ptr | const char | ptr | Const is associated with data type ,so value is constant |
char * const ptr | char | const ptr | Const is associated with pointer ,so pointer is constant |
const char * const ptr | const char | const ptr | Const is associated with both data type & pointer so both are constant |
Conclusion
In conclusion, understanding the differences between pointer constants and constant pointers empowers C programmers to work with pointers more effectively. We’ve seen how these concepts are useful in various situations, including C programming, especially when programming Embedded Systems.
Having a good grasp of pointer constants and constant pointers takes your C programming skills to a higher level. With this knowledge, programmers can write code that is efficient, secure, and dependable, fully tapping into the capabilities of pointers in their projects.
This expertise allows programmers to manage memory more efficiently by allocating and releasing it appropriately, which in turn improves the program’s performance and prevents memory-related issues. By using constant pointers properly, programmers can also prevent accidental changes to important memory locations, making their software more stable and less prone to vulnerabilities caused by unintended data modifications.
In summary, mastering pointers not only simplifies working with pointers in C but also enhances a programmer’s overall skill set. Armed with this knowledge, programmers can create solutions that combine reliability and efficiency, unlocking the full potential of pointers in all their programming endeavors.
If you’re looking to enhance your skills in C programming, our comprehensive guide on Emertxe’s Online Training Programs in Embedded Systems & IoT can provide valuable insights and resources.
SN | Blogs | Links |
---|---|---|
1. | Enumeration in C | Click Here |
2. | Type Promotion in C | Click Here |
3. | Difference between typedef and macro in C | Click Here |
People Also Ask(PAA)
If you attempt to modify the address stored in a constant pointer, you’ll encounter a compilation error. Constant pointers are designed to have a fixed memory address, which means that once they are assigned a specific address, that address cannot be changed. Any attempt to reassign a new address to a constant pointer will result in a compilation error, indicating that the pointer is read-only and its address cannot be modified.
Pointer constants contribute to the clarity of the code by indicating that the memory address won’t change, enhancing readability, and clarifying intentions. Contribution of constant pointer is it Increase code maintainability by preventing accidental reassignment, reducing the risk of errors and improving long-term stability.
Advantages of using pointer constants In order to facilitate predictable hardware interactions, ensure memory address stability. Constant pointers provide the advantage of protecting against unintentional modifications and improving code stability.Together, they advocate for reliable, effective code for embedded systems that are resource-constrained.