Introduction
In embedded systems, efficient memory management is crucial, especially when dealing with various types of sensors that provide data in different formats. A real-world example is a weather station that collects data from temperature, humidity, and pressure sensors. Each of these sensors outputs data in a unique format, which poses a challenge for efficient storage and processing.
This blog post explores how unions in C can be used to manage sensor data efficiently. By using a union, we can save memory and handle different data types within the same structure. We’ll walk through a practical example where sensor data is read, processed, and stored in a memory-optimized way, demonstrating how unions are a key tool for embedded system applications.
Manage Sensor Data in an Embedded System
This example will focus on a scenario where an embedded system needs to read data from multiple types of sensors and handle each type differently while saving memory.
Real-World Sensor Application in C
Scenario:
Imagine a weather station that collects data from different sensors: temperature, humidity, and pressure. Each sensor provides data in a different format. The goal is to read the data from each sensor, process it accordingly, and store it efficiently.
Objective:
Use a union to manage memory efficiently while handling different data types for each sensor.
Sensor Data Structure
- Temperature Sensor: Provides data as an
int
representing temperature in Celsius. - Humidity Sensor: Provides data as a
float
representing the percentage of humidity. - Pressure Sensor: Provides data as a
long
representing pressure in Pascals.
Code Example
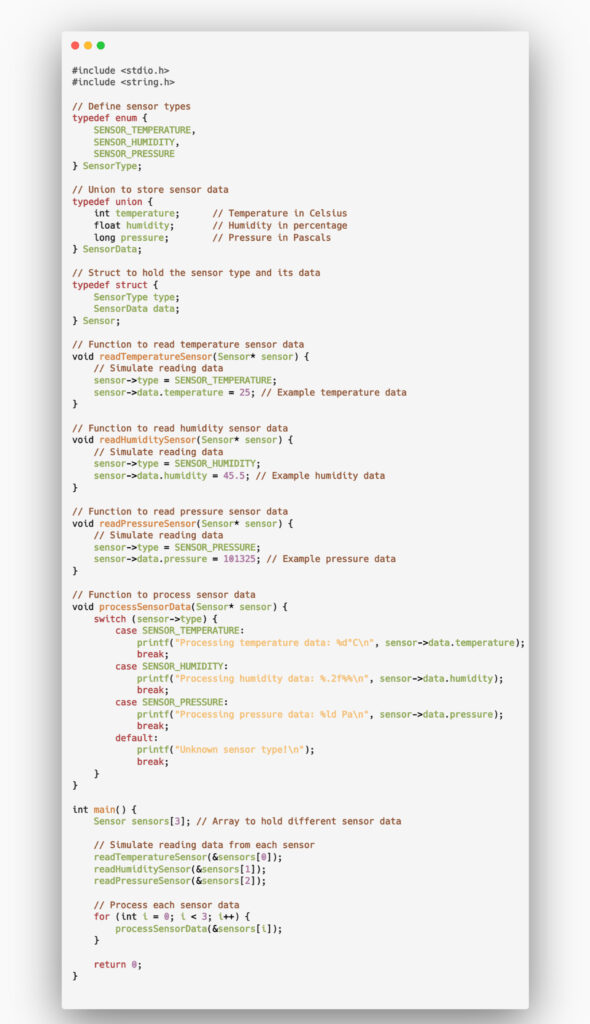
Output:
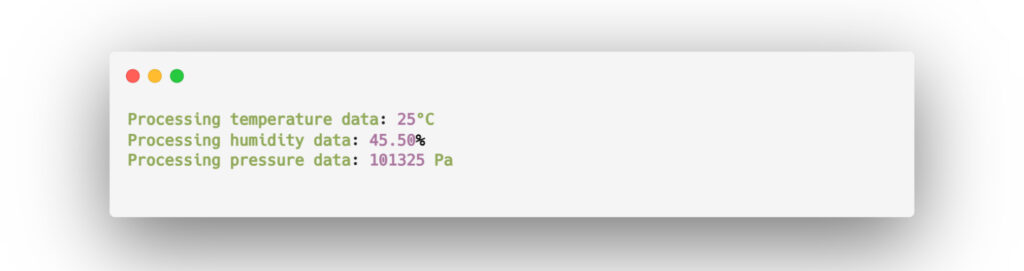
Explanation
Sensor Type Enumeration (
SensorType
):- Defines the types of sensors available (temperature, humidity, pressure).
Union (
SensorData
):- Holds the sensor data. It can store an
int
,float
, orlong
, depending on the sensor type. This union allows all sensor data to share the same memory space, conserving memory.
- Holds the sensor data. It can store an
Struct (
Sensor
):- Combines the sensor type and the union holding the data. This structure enables the program to manage the sensor data efficiently by knowing the type and the associated data format.
Reading Functions:
- Functions like
readTemperatureSensor
,readHumiditySensor
, andreadPressureSensor
simulate the reading of sensor data and store the result in theSensor
structure.
- Functions like
Processing Function (
processSensorData
):- This function processes the sensor data based on its type, demonstrating how to handle different data types stored in a union.
Key Benefits
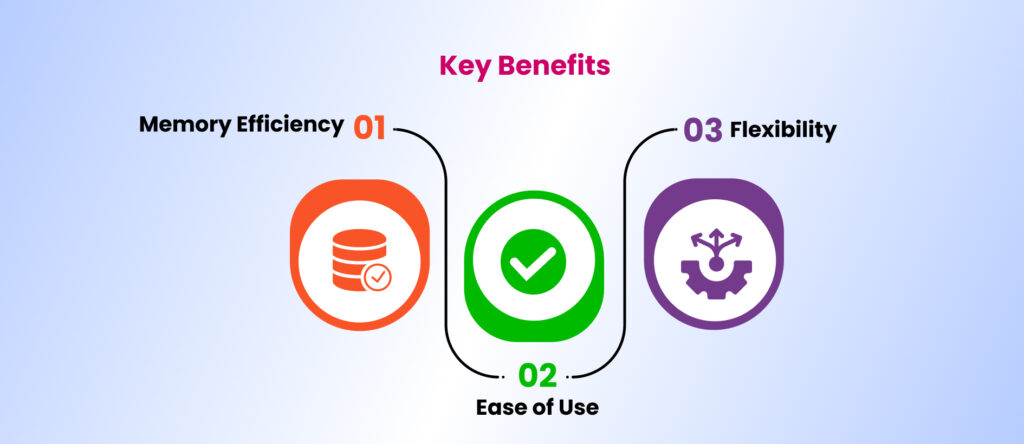
- Memory Efficiency: By using a union, the program only allocates memory for the largest data type (in this case,
long
for pressure), regardless of the actual sensor type being stored. This is especially useful in memory-constrained environments like embedded systems. - Ease of Use: The structure simplifies managing different sensor types, allowing the program to easily switch between handling various sensors based on the
type
field. - Flexibility: The union and struct combination allows for easy expansion and modification if new sensor types or data formats are introduced.
Real Application Context
In an actual embedded system:
- The functions readTemperatureSensor, readHumiditySensor, and readPressureSensor would interface with hardware sensors to collect real data.
- The
processSensorData
function might perform more complex operations, such as sending data to a remote server, logging it for later analysis, or triggering alarms if the sensor data is out of a specified range.
This example showcases how unions can be effectively used in real-world applications to handle diverse data types while optimizing for memory usage and processing efficiency.
Conclusion
Unions in C provide an efficient way to manage different data types in memory-constrained environments, such as embedded systems. In our weather station example, we saw how a union allows sensor data from temperature, humidity, and pressure sensors to share the same memory space, reducing memory usage while still enabling precise data handling. This approach simplifies sensor data management and offers flexibility for adding new sensor types in the future.
By combining unions with structures, we not only save memory but also maintain code clarity and ease of use. In real-world embedded applications, where memory is limited and performance is critical, unions are a powerful tool for optimizing both memory and processing efficiency.
Serial No. | Related Blogs | Links |
---|---|---|
1. | Why is C The Most Preferred Language for Embedded Systems? | Click Here |
2. | Future Trends in IoT: Insights from Bangalore’s Tech Experts | Click Here |
3. | Internet-Of-Things (IoT) in Healthcare – The Critical Factor | Click Here |
People Also Ask (PAA)
Unions can be used in a variety of situations where memory optimization is crucial. For example, they are used in network protocols to store different types of packet data, or in embedded devices where different states or modes need to be represented with minimal memory overhead.
Since unions don’t track which member currently holds data, programmers usually include a separate variable (such as an enum or an identifier) to track the type of data stored. In the sensor example, the SensorType
enum helps track whether the union contains temperature, humidity, or pressure data.
Yes, unions can store complex data types such as arrays, structures, or even other unions. However, only one of these members can hold a value at a time. This flexibility makes unions useful for representing different kinds of complex data while conserving memory.
Unions are widely used in embedded systems because they help optimize memory usage. In environments where memory is limited, such as microcontrollers, unions allow for efficient handling of data by sharing the same memory for different types of variables, which is crucial in managing sensor data, device states, and configurations.